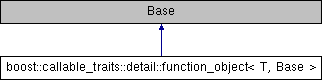
Documentation
template<typename T, typename Base>
struct boost::callable_traits::detail::function_object< T, Base >
Definition at line 20 of file function_object.hpp.
Public Types | |
using | type = T |
using | error_t = error_type< T > |
using | function_type = typename Base::function_object_signature |
using | arg_types = typename Base::non_invoke_arg_types |
using | non_invoke_arg_types = arg_types |
using | traits = function_object |
using | class_type = error_t |
using | invoke_type = error_t |
using | remove_varargs = error_t |
using | add_varargs = error_t |
using | is_noexcept = typename Base::is_noexcept |
using | add_noexcept = error_t |
using | remove_noexcept = error_t |
using | is_transaction_safe = typename Base::is_transaction_safe |
using | add_transaction_safe = error_t |
using | remove_transaction_safe = error_t |
using | clear_args = error_t |
template<template< class... > class Container> | |
using | expand_args = typename function< function_type >::template expand_args< Container > |
template<template< class... > class Container, typename... RightArgs> | |
using | expand_args_left = typename function< function_type >::template expand_args_left< Container, RightArgs... > |
template<template< class... > class Container, typename... LeftArgs> | |
using | expand_args_right = typename function< function_type >::template expand_args_right< Container, LeftArgs... > |
template<typename C , typename U = T> | |
using | apply_member_pointer = typename std::remove_reference< U >::type C::* |
template<typename > | |
using | apply_return = error_t |
template<typename... > | |
using | push_front = error_t |
template<typename... > | |
using | push_back = error_t |
template<std::size_t ElementCount> | |
using | pop_args_front = error_t |
template<std::size_t ElementCount> | |
using | pop_args_back = error_t |
template<std::size_t Index, typename... NewArgs> | |
using | insert_args = error_t |
template<std::size_t Index, std::size_t Count> | |
using | remove_args = error_t |
template<std::size_t Index, typename... NewArgs> | |
using | replace_args = error_t |
template<std::size_t Count> | |
using | pop_front = error_t |
template<std::size_t Count> | |
using | pop_back = error_t |
using | remove_member_reference = error_t |
using | add_member_lvalue_reference = error_t |
using | add_member_rvalue_reference = error_t |
using | add_member_const = error_t |
using | add_member_volatile = error_t |
using | add_member_cv = error_t |
using | remove_member_const = error_t |
using | remove_member_volatile = error_t |
using | remove_member_cv = error_t |
Static Public Attributes | |
static constexpr const bool | value |
Member Typedef Documentation
◆ add_member_const
using boost::callable_traits::detail::function_object< T, Base >::add_member_const = error_t |
Definition at line 93 of file function_object.hpp.
◆ add_member_cv
using boost::callable_traits::detail::function_object< T, Base >::add_member_cv = error_t |
Definition at line 95 of file function_object.hpp.
◆ add_member_lvalue_reference
using boost::callable_traits::detail::function_object< T, Base >::add_member_lvalue_reference = error_t |
Definition at line 91 of file function_object.hpp.
◆ add_member_rvalue_reference
using boost::callable_traits::detail::function_object< T, Base >::add_member_rvalue_reference = error_t |
Definition at line 92 of file function_object.hpp.
◆ add_member_volatile
using boost::callable_traits::detail::function_object< T, Base >::add_member_volatile = error_t |
Definition at line 94 of file function_object.hpp.
◆ add_noexcept
using boost::callable_traits::detail::function_object< T, Base >::add_noexcept = error_t |
Definition at line 37 of file function_object.hpp.
◆ add_transaction_safe
using boost::callable_traits::detail::function_object< T, Base >::add_transaction_safe = error_t |
Definition at line 40 of file function_object.hpp.
◆ add_varargs
using boost::callable_traits::detail::function_object< T, Base >::add_varargs = error_t |
Definition at line 35 of file function_object.hpp.
◆ apply_member_pointer
using boost::callable_traits::detail::function_object< T, Base >::apply_member_pointer = typename std::remove_reference<U>::type C::* |
Definition at line 57 of file function_object.hpp.
◆ apply_return
using boost::callable_traits::detail::function_object< T, Base >::apply_return = error_t |
Definition at line 61 of file function_object.hpp.
◆ arg_types
using boost::callable_traits::detail::function_object< T, Base >::arg_types = typename Base::non_invoke_arg_types |
Definition at line 25 of file function_object.hpp.
◆ class_type
using boost::callable_traits::detail::function_object< T, Base >::class_type = error_t |
Definition at line 32 of file function_object.hpp.
◆ clear_args
using boost::callable_traits::detail::function_object< T, Base >::clear_args = error_t |
Definition at line 42 of file function_object.hpp.
◆ error_t
using boost::callable_traits::detail::function_object< T, Base >::error_t = error_type<T> |
Definition at line 23 of file function_object.hpp.
◆ expand_args
using boost::callable_traits::detail::function_object< T, Base >::expand_args = typename function<function_type>::template expand_args<Container> |
Definition at line 45 of file function_object.hpp.
◆ expand_args_left
using boost::callable_traits::detail::function_object< T, Base >::expand_args_left = typename function<function_type>::template expand_args_left<Container, RightArgs...> |
Definition at line 49 of file function_object.hpp.
◆ expand_args_right
using boost::callable_traits::detail::function_object< T, Base >::expand_args_right = typename function<function_type>::template expand_args_right<Container, LeftArgs...> |
Definition at line 53 of file function_object.hpp.
◆ function_type
using boost::callable_traits::detail::function_object< T, Base >::function_type = typename Base::function_object_signature |
Definition at line 24 of file function_object.hpp.
◆ insert_args
using boost::callable_traits::detail::function_object< T, Base >::insert_args = error_t |
Definition at line 76 of file function_object.hpp.
◆ invoke_type
using boost::callable_traits::detail::function_object< T, Base >::invoke_type = error_t |
Definition at line 33 of file function_object.hpp.
◆ is_noexcept
using boost::callable_traits::detail::function_object< T, Base >::is_noexcept = typename Base::is_noexcept |
Definition at line 36 of file function_object.hpp.
◆ is_transaction_safe
using boost::callable_traits::detail::function_object< T, Base >::is_transaction_safe = typename Base::is_transaction_safe |
Definition at line 39 of file function_object.hpp.
◆ non_invoke_arg_types
using boost::callable_traits::detail::function_object< T, Base >::non_invoke_arg_types = arg_types |
Definition at line 26 of file function_object.hpp.
◆ pop_args_back
using boost::callable_traits::detail::function_object< T, Base >::pop_args_back = error_t |
Definition at line 73 of file function_object.hpp.
◆ pop_args_front
using boost::callable_traits::detail::function_object< T, Base >::pop_args_front = error_t |
Definition at line 70 of file function_object.hpp.
◆ pop_back
using boost::callable_traits::detail::function_object< T, Base >::pop_back = error_t |
Definition at line 88 of file function_object.hpp.
◆ pop_front
using boost::callable_traits::detail::function_object< T, Base >::pop_front = error_t |
Definition at line 85 of file function_object.hpp.
◆ push_back
using boost::callable_traits::detail::function_object< T, Base >::push_back = error_t |
Definition at line 67 of file function_object.hpp.
◆ push_front
using boost::callable_traits::detail::function_object< T, Base >::push_front = error_t |
Definition at line 64 of file function_object.hpp.
◆ remove_args
using boost::callable_traits::detail::function_object< T, Base >::remove_args = error_t |
Definition at line 79 of file function_object.hpp.
◆ remove_member_const
using boost::callable_traits::detail::function_object< T, Base >::remove_member_const = error_t |
Definition at line 96 of file function_object.hpp.
◆ remove_member_cv
using boost::callable_traits::detail::function_object< T, Base >::remove_member_cv = error_t |
Definition at line 98 of file function_object.hpp.
◆ remove_member_reference
using boost::callable_traits::detail::function_object< T, Base >::remove_member_reference = error_t |
Definition at line 90 of file function_object.hpp.
◆ remove_member_volatile
using boost::callable_traits::detail::function_object< T, Base >::remove_member_volatile = error_t |
Definition at line 97 of file function_object.hpp.
◆ remove_noexcept
using boost::callable_traits::detail::function_object< T, Base >::remove_noexcept = error_t |
Definition at line 38 of file function_object.hpp.
◆ remove_transaction_safe
using boost::callable_traits::detail::function_object< T, Base >::remove_transaction_safe = error_t |
Definition at line 41 of file function_object.hpp.
◆ remove_varargs
using boost::callable_traits::detail::function_object< T, Base >::remove_varargs = error_t |
Definition at line 34 of file function_object.hpp.
◆ replace_args
using boost::callable_traits::detail::function_object< T, Base >::replace_args = error_t |
Definition at line 82 of file function_object.hpp.
◆ traits
using boost::callable_traits::detail::function_object< T, Base >::traits = function_object |
Definition at line 31 of file function_object.hpp.
◆ type
using boost::callable_traits::detail::function_object< T, Base >::type = T |
Definition at line 22 of file function_object.hpp.
Member Data Documentation
◆ value
|
staticconstexpr |
Definition at line 28 of file function_object.hpp.
The documentation for this struct was generated from the following files:
- ttg/ttg/external/boost/callable_traits/detail/forward_declarations.hpp
- ttg/ttg/external/boost/callable_traits/detail/function_object.hpp