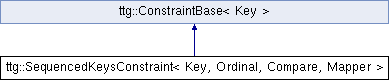
Documentation
template<typename Key, typename Ordinal = std::size_t, typename Compare = std::less<Ordinal>, typename Mapper = ttg::Void>
struct ttg::SequencedKeysConstraint< Key, Ordinal, Compare, Mapper >
Definition at line 70 of file constraint.h.
Classes | |
struct | sequence_elem_t |
Public Types | |
using | key_type = std::conditional_t< ttg::meta::is_void_v< Key >, ttg::Void, Key > |
using | ordinal_type = Ordinal |
using | keymap_t = std::function< Ordinal(const key_type &)> |
using | compare_t = Compare |
using | base_t = ConstraintBase< Key > |
![]() | |
using | key_type = Key |
using | listener_t = std::function< void(const ttg::span< key_type > &)> |
Public Member Functions | |
SequencedKeysConstraint (bool auto_release=false) | |
template<typename Mapper_ > | |
requires (std::is_invocable_v< Mapper_, Key >) SequencedKeysConstraint(Mapper_ &&map | |
SequencedKeysConstraint (const SequencedKeysConstraint &skc)=default | |
SequencedKeysConstraint & | operator= (SequencedKeysConstraint &&skc)=default |
SequencedKeysConstraint & | operator= (const SequencedKeysConstraint &skc)=default |
virtual | ~SequencedKeysConstraint ()=default |
template<typename Key_ = key_type, typename Mapper_ = Mapper> | |
std::enable_if_t<!ttg::meta::is_void_v< Key_ > &&!ttg::meta::is_void_v< Mapper_ >, bool > | check (const key_type &key, ttg::TTBase *tt) |
template<typename Key_ = key_type, typename Mapper_ = Mapper> | |
std::enable_if_t<!ttg::meta::is_void_v< Key_ > &&ttg::meta::is_void_v< Mapper_ >, bool > | check (const key_type &key, Ordinal ord, ttg::TTBase *tt) |
template<typename Key_ = key_type, typename Mapper_ = Mapper> | |
std::enable_if_t< ttg::meta::is_void_v< Key_ > &&!ttg::meta::is_void_v< Mapper_ >, bool > | check (ttg::TTBase *tt) |
template<typename Key_ = key_type, typename Mapper_ = Mapper> | |
std::enable_if_t< ttg::meta::is_void_v< Key_ > &&ttg::meta::is_void_v< Mapper_ >, bool > | check (ordinal_type ord, ttg::TTBase *tt) |
template<typename Key_ = key_type, typename Mapper_ = Mapper> | |
std::enable_if_t<!ttg::meta::is_void_v< Key_ > &&!ttg::meta::is_void_v< Mapper_ > > | complete (const key_type &key, ttg::TTBase *tt) |
template<typename Key_ = key_type, typename Mapper_ = Mapper> | |
std::enable_if_t<!ttg::meta::is_void_v< Key_ > &&ttg::meta::is_void_v< Mapper_ > > | complete (const key_type &key, Ordinal ord, ttg::TTBase *tt) |
template<typename Key_ = key_type, typename Mapper_ = Mapper> | |
std::enable_if_t<!ttg::meta::is_void_v< Key_ > &&ttg::meta::is_void_v< Mapper_ > > | complete (Ordinal ord, ttg::TTBase *tt) |
template<typename Key_ = key_type, typename Mapper_ = Mapper> | |
std::enable_if_t<!ttg::meta::is_void_v< Key_ > &&!ttg::meta::is_void_v< Mapper_ > > | complete (ttg::TTBase *tt) |
void | stop () |
void | start () |
void | release (ordinal_type ord=0) |
bool | is_auto () const |
![]() | |
ConstraintBase () | |
ConstraintBase (ConstraintBase &&cb) | |
ConstraintBase (const ConstraintBase &cb) | |
ConstraintBase & | operator= (ConstraintBase &&cb) |
ConstraintBase & | operator= (const ConstraintBase &cb) |
virtual | ~ConstraintBase ()=default |
void | add_listener (listener_t l, ttg::TTBase *tt) |
void | notify_listener (const ttg::span< key_type > &keys, ttg::TTBase *tt) |
Public Attributes | |
bool | auto_release |
Protected Member Functions | |
bool | comp_equal (const Ordinal &a, const Ordinal &b) const |
bool | eligible (const Ordinal &ord) const |
bool | check_key_impl (const key_type &key, Ordinal ord, ttg::TTBase *tt) |
void | complete_key_impl () |
void | release_next () |
void | release_next (ordinal_type ord, bool force_check=false) |
![]() | |
auto | lock_guard () |
Protected Attributes | |
std::map< ordinal_type, sequence_elem_t, compare_t > | m_sequence |
ordinal_type | m_current = std::numeric_limits<ordinal_type>::min() |
Mapper | m_map |
compare_t | m_order |
std::atomic< std::size_t > | m_active |
bool | m_stopped = false |
bool | m_auto_release = false |
Member Typedef Documentation
◆ base_t
using ttg::SequencedKeysConstraint< Key, Ordinal, Compare, Mapper >::base_t = ConstraintBase<Key> |
Definition at line 76 of file constraint.h.
◆ compare_t
using ttg::SequencedKeysConstraint< Key, Ordinal, Compare, Mapper >::compare_t = Compare |
Definition at line 75 of file constraint.h.
◆ key_type
using ttg::SequencedKeysConstraint< Key, Ordinal, Compare, Mapper >::key_type = std::conditional_t<ttg::meta::is_void_v<Key>, ttg::Void, Key> |
Definition at line 72 of file constraint.h.
◆ keymap_t
using ttg::SequencedKeysConstraint< Key, Ordinal, Compare, Mapper >::keymap_t = std::function<Ordinal(const key_type&)> |
Definition at line 74 of file constraint.h.
◆ ordinal_type
using ttg::SequencedKeysConstraint< Key, Ordinal, Compare, Mapper >::ordinal_type = Ordinal |
Definition at line 73 of file constraint.h.
Constructor & Destructor Documentation
◆ SequencedKeysConstraint() [1/2]
|
inline |
Used for external key mapper.
Definition at line 219 of file constraint.h.
◆ SequencedKeysConstraint() [2/2]
|
default |
◆ ~SequencedKeysConstraint()
|
virtualdefault |
Member Function Documentation
◆ check() [1/4]
|
inline |
Definition at line 254 of file constraint.h.
◆ check() [2/4]
|
inline |
Definition at line 247 of file constraint.h.
◆ check() [3/4]
|
inline |
Definition at line 266 of file constraint.h.
◆ check() [4/4]
|
inline |
Definition at line 260 of file constraint.h.
◆ check_key_impl()
|
inlineprotected |
Definition at line 108 of file constraint.h.
◆ comp_equal()
|
inlineprotected |
Definition at line 100 of file constraint.h.
◆ complete() [1/4]
|
inline |
Definition at line 278 of file constraint.h.
◆ complete() [2/4]
|
inline |
Definition at line 272 of file constraint.h.
◆ complete() [3/4]
|
inline |
Definition at line 284 of file constraint.h.
◆ complete() [4/4]
|
inline |
Definition at line 290 of file constraint.h.
◆ complete_key_impl()
|
inlineprotected |
Definition at line 145 of file constraint.h.
◆ eligible()
|
inlineprotected |
Definition at line 104 of file constraint.h.
◆ is_auto()
|
inline |
Definition at line 338 of file constraint.h.
◆ operator=() [1/2]
|
default |
◆ operator=() [2/2]
|
default |
◆ release()
|
inline |
Release tasks up to the ordinal. The provided ordinal is ignored if auto_release
is enabled.
Definition at line 328 of file constraint.h.
◆ release_next() [1/2]
|
inlineprotected |
Definition at line 155 of file constraint.h.
◆ release_next() [2/2]
|
inlineprotected |
Definition at line 183 of file constraint.h.
◆ requires()
ttg::SequencedKeysConstraint< Key, Ordinal, Compare, Mapper >::requires | ( | std::is_invocable_v< Mapper_, Key > | ) | && |
◆ start()
|
inline |
Start execution. This constraint is not stopped by default so calls to start
are only necessary if explictily stopped.
Definition at line 308 of file constraint.h.
◆ stop()
|
inline |
Stop all execution. Call start
to resume. This constraint is not stopped by default so calls to start
are only necessary if explictily stopped.
Definition at line 299 of file constraint.h.
Member Data Documentation
◆ auto_release
bool ttg::SequencedKeysConstraint< Key, Ordinal, Compare, Mapper >::auto_release |
Definition at line 226 of file constraint.h.
◆ m_active
|
protected |
Definition at line 350 of file constraint.h.
◆ m_auto_release
|
protected |
Definition at line 352 of file constraint.h.
◆ m_current
|
protected |
Definition at line 345 of file constraint.h.
◆ m_map
|
protected |
Definition at line 347 of file constraint.h.
◆ m_order
|
protected |
Definition at line 349 of file constraint.h.
◆ m_sequence
|
protected |
Definition at line 344 of file constraint.h.
◆ m_stopped
|
protected |
Definition at line 351 of file constraint.h.
The documentation for this struct was generated from the following file:
- ttg/ttg/constraint.h