ttg_madness::Buffer< T, Allocator > Struct Template Reference
Inheritance diagram for ttg_madness::Buffer< T, Allocator >:
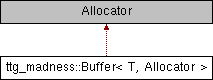
Documentation
template<typename T, typename Allocator>
struct ttg_madness::Buffer< T, Allocator >
A runtime-managed buffer mirrored between host and device memory.
Public Types | |
using | element_type = std::decay_t< T > |
using | allocator_traits = std::allocator_traits< Allocator > |
using | allocator_type = typename allocator_traits::allocator_type |
Member Typedef Documentation
◆ allocator_traits
template<typename T , typename Allocator >
using ttg_madness::Buffer< T, Allocator >::allocator_traits = std::allocator_traits<Allocator> |
◆ allocator_type
template<typename T , typename Allocator >
using ttg_madness::Buffer< T, Allocator >::allocator_type = typename allocator_traits::allocator_type |
◆ element_type
template<typename T , typename Allocator >
using ttg_madness::Buffer< T, Allocator >::element_type = std::decay_t<T> |
Constructor & Destructor Documentation
◆ Buffer() [1/5]
template<typename T , typename Allocator >
|
inline |
◆ Buffer() [2/5]
template<typename T , typename Allocator >
|
inline |
◆ Buffer() [3/5]
template<typename T , typename Allocator >
|
inline |
◆ ~Buffer()
template<typename T , typename Allocator >
|
inlinevirtual |
◆ Buffer() [4/5]
template<typename T , typename Allocator >
|
inline |
◆ Buffer() [5/5]
template<typename T , typename Allocator >
|
delete |
Member Function Documentation
◆ allocate_on()
template<typename T , typename Allocator >
|
inline |
◆ current_device_ptr() [1/2]
template<typename T , typename Allocator >
|
inline |
◆ current_device_ptr() [2/2]
template<typename T , typename Allocator >
|
inline |
◆ device_ptr_on() [1/2]
template<typename T , typename Allocator >
|
inline |
◆ device_ptr_on() [2/2]
template<typename T , typename Allocator >
|
inline |
◆ get_owner_device()
template<typename T , typename Allocator >
|
inline |
◆ host_ptr() [1/2]
template<typename T , typename Allocator >
|
inline |
◆ host_ptr() [2/2]
template<typename T , typename Allocator >
|
inline |
◆ is_valid()
template<typename T , typename Allocator >
|
inline |
◆ is_valid_on()
template<typename T , typename Allocator >
|
inline |
◆ operator bool()
template<typename T , typename Allocator >
|
inline |
◆ operator=() [1/2]
template<typename T , typename Allocator >
|
inline |
◆ operator=() [2/2]
template<typename T , typename Allocator >
|
delete |
◆ owner_device_ptr() [1/2]
template<typename T , typename Allocator >
|
inline |
◆ owner_device_ptr() [2/2]
template<typename T , typename Allocator >
|
inline |
◆ pin()
template<typename T , typename Allocator >
|
inline |
◆ pin_on()
template<typename T , typename Allocator >
|
inline |
◆ reset() [1/2]
template<typename T , typename Allocator >
|
inline |
◆ reset() [2/2]
template<typename T , typename Allocator >
|
inline |
◆ set_current_device()
template<typename T , typename Allocator >
|
inline |
◆ size()
template<typename T , typename Allocator >
|
inline |
◆ unpin()
template<typename T , typename Allocator >
|
inline |
◆ unpin_on()
template<typename T , typename Allocator >
|
inline |
The documentation for this struct was generated from the following file:
- ttg/ttg/madness/buffer.h